A string prefix is a character(s) at the beginning of a string.
For Example: in apple, app or ap or apps etc are prefix
Problem
Find the common longest prefix for strings apple, apps and appster
Approach
- Sort all the string in alphabetical order.
- Find the small string
- Loop through the small string by each character.
- Compare each character in other string at same index.
- If in any iteration there is no match of any character at any index, Exit.
- String of all character before #5 is your common prefix
Code
import java.util.ArrayList;
import java.util.List;
public class PrefixFinder {
public static void main(String[] args) {
List<String> fruits = new ArrayList<String>();
fruits.add("apple");
fruits.add("apps");
fruits.add("appster");
String str = fruits.get(0);
//find the short value
for(String fruit: fruits) {
if (str.length() > fruit.length()) {
str = fruit;
}
}
System.out.println("small string is " + str);
String pref = "";
Boolean flag = false;
for(int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
for(int j=0; j<fruits.size(); j++) {
if(fruits.get(j).charAt(i) != c) {
flag = true;
break;
}
}
if(flag == false) {
pref = pref + c;
}
}
System.out.println("prefix is " + pref);
}
}
Run Code
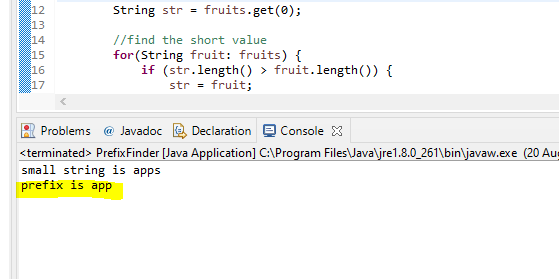