In mathematics Fibonacci Sequence is the series of numbers as given below
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, …
The next number is found by adding up the two numbers before it:
For Example : In above series 2 at index 4 is found by adding the two numbers before it (1+1)
3 at index 5 is found by adding the two numbers before it (1+2)
5 at index 6 is found by adding the two numbers before it (2+3)
and so on!
Problem
write a java code for Fibonacci series or Fibonacci sequence or Fibonacci number
Approach
- Get the number using scanner stdin
- Create a function which return the sum of number before two numbers
- if number is 1 or 2 then return 1
- else return sum of last two numbers
Code
import java.util.Scanner;
class Fibonacci {
public static int fibonacci(Integer num) {
if(num == 1 || num ==2) {
return 1;
}
return fibonacci(num-2 ) + fibonacci(num-1);
}
public static void main(String[] args) {
System.out.println("Enter no ");
Scanner scanner = new Scanner(System.in);
int cnt = scanner.nextInt();
scanner.close();
for(int i=1; i<=cnt; i++ ) {
System.out.print(Fibonacci.fibonacci(i) + " ");
}
}
}
Run Code
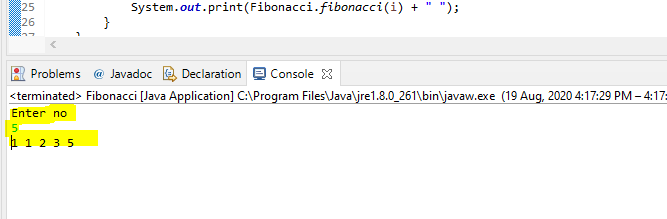