Armstrong number is a number that is equal to the sum of cubes of its digits.
For example 0, 1, 153, 370, 371 and 407 are the Armstrong numbers. let take an example of the armstrong number 153.
Sum of the cube of each digit is equal to the same number.
153 = 1^3 + 5^3 + 3^3;
Code Problem
Write a java code for the armstrong number.
Approach
- Get the input using the Scanner stdin.
- Run a while loop unless the number is greater than zero.
- Extract each number.
- Find the cube of each number and sum the value.
import java.util.Scanner;
public class Armstrong {
public static void arm(int num) {
int c=0;
int temp;
int a;
temp=num;
while(num > 0) {
a = num % 10;
c = c+(a*a*a);
num = num / 10;
}
if(temp == c ){
System.out.println("Armstrong");
} else {
System.out.println("Not Armstrong");
}
}
public static void main(String[] args) {
System.out.println("Enter number ");
Scanner scanner = new Scanner(System.in);
int cnt = scanner.nextInt();
scanner.close();
Armstrong.arm(cnt);
}
}
Run Code
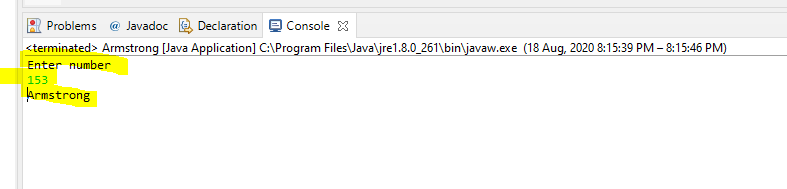